Working with Nested Dictionaries
Learn how to work with nested dictionaries in Jellycuts with this step-by-step guide.
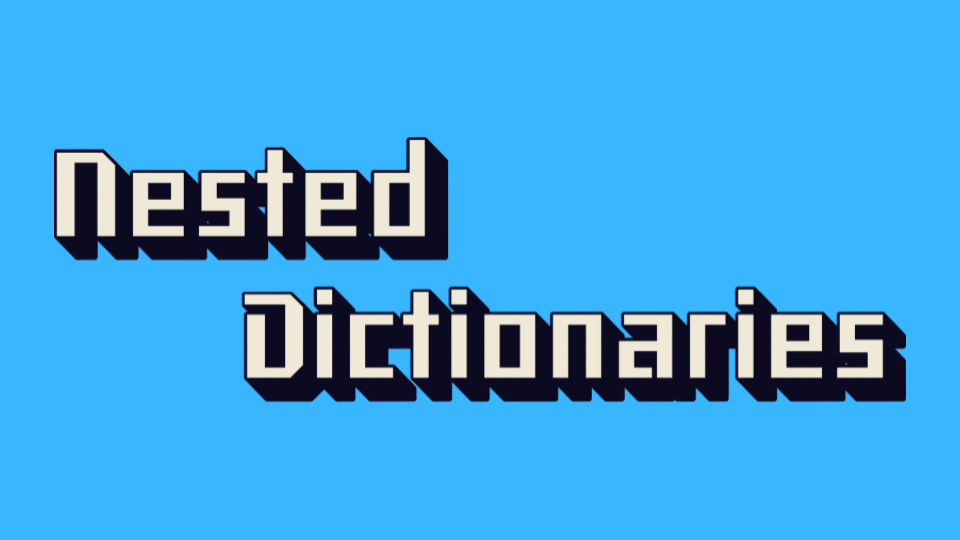
Jellycuts is a powerful tool for automating tasks on iOS devices using custom shortcuts. Understanding how to work with nested dictionaries in Jellycuts can be incredibly useful for managing complex data structures. This guide will walk you through a full example of working with nested dictionaries, breaking it down step-by-step.
Full Code Example
The example below is loosely based on my previous article where I share how I version control Shortcuts on RoutineHub using UpdateKit. I've modified it slightly so that the JSON-based Dictionary is obviously nested.
If you would like to see this the final example, download the Shortcut from RoutineHub.
import Shortcuts
#Color: red, #Icon: shortcuts
// JSON Dictionary I use to Version Control
dictionary(json: { "routinehub": { "author": { "name": "0xChris", "url": "https://routinehub.co/user/0xchris" }, "shortcut": { "name": "0xQRMaker", "id": "18187", "url": "https://routinehub.co/shortcut/18187/"}} }) >> casestudy
// ———— Top Level JSON ——
valueFor(key: "routinehub", dictionary: casestudy) >> rhDict
// Show Items within "routinehub"
keysFrom(dictionary: rhDict) >> rhKeys
quicklook(input: rhKeys)
// ———— About Author Node ——————
// These two approaches aims to get the precise value you need
// Get Value using .key() method
var authorName = rhDict.key(author).key(name)
quicklook(input: authorName)
// Get Value using Interpolation
var authorURL = "${rhDict.as(Dictionary).key(author).as(Dictionary).key(url)}"
quicklook(input: authorURL)
// ———— About Shortcut ——————
// This strategy aims to first identify what keys are available for future targeting
// Get Items within "shortcut"
valueFor(key: "shortcut", dictionary: rhDict) >> shortcutDict
// Get keys of items within "shortcut"
keysFrom(dictionary: shortcutDict) >> shortcutKeys
quicklook(input: shortcutKeys)
// Get value from shortcut.id
var shortcutID = shortcutDict.key(id)
quicklook(input: shortcutID)
// Get value from shortcut.name
valueFor(key: "name", dictionary: shortcutDict) >> name
quicklook(input: name)
This JSON is simply meant to keep record of my shortcut and what version I am using.
Breaking It Down
Let's break down the code into smaller, more understandable parts.
Step 1: Creating the Dictionary
We start by creating a dictionary (a collection of key-value pairs) in JSON format. This dictionary contains information about me, the author, and my shortcut.
dictionary(json: { "routinehub": { "author": { "name": "0xChris", "url": "https://routinehub.co/user/0xchris" }, "shortcut": { "name": "0xQRMaker", "id": "18187", "url": "https://routinehub.co/shortcut/18187/" }}
}) >> casestudy
This JSON is simply meant to keep record of my shortcut and what version I am using.
Step 2: Accessing the Top-Level Dictionary
We extract the top-level dictionary using the key "routinehub".
valueFor(key: "routinehub", dictionary: casestudy) >> rhDict
Step 3: Listing Keys in the Top-Level Dictionary
We list all the keys in the "routinehub" dictionary to see what it contains.
keysFrom(dictionary: rhDict) >> rhKeys
quicklook(input: rhKeys)
The quicklook
command will show the keys in the "routinehub" dictionary: ["author", "shortcut"]
.
Step 4: Accessing Values in the "Author" Node
Method 1: Using the .key()
Method
We get the author's name using the .key()
method.
var authorName = rhDict.key(author).key(name)
quicklook(input: authorName)
This will display 0xChris
.
Method 2: Using Interpolation
We get the author's URL using string interpolation.
var authorURL = "${rhDict.as(Dictionary).key(author).as(Dictionary).key(url)}"
quicklook(input: authorURL)
This will display https://routinehub.co/user/0xchris
.
Step 5: Accessing Values in the "Shortcut" Node
Step 5.1: Extracting the "Shortcut" Dictionary
We extract the "shortcut" dictionary from the "routinehub" dictionary.
valueFor(key: "shortcut", dictionary: rhDict) >> shortcutDict
Step 5.2: Listing Keys in the "Shortcut" Dictionary
We list all the keys in the "shortcut" dictionary.
keysFrom(dictionary: shortcutDict) >> shortcutKeys
quicklook(input: shortcutKeys)
The quicklook
command will show the keys in the "shortcut" dictionary: ["name", "id", "url"]
.
Step 5.3: Accessing Specific Values in the "Shortcut" Dictionary
We get the shortcut ID.
var shortcutID = shortcutDict.key(id)
quicklook(input: shortcutID)
This will display 18187
.
We get the shortcut name.
valueFor(key: "name", dictionary: shortcutDict) >> name
quicklook(input: name)
This will display 0xQRMaker
.
Conclusion
By following these steps, you can effectively work with nested dictionaries in Jellycuts. This approach allows you to access and manipulate complex data structures with ease, making your shortcuts more powerful and versatile.