How to Send a GET Request in Jellycuts
Learn how to send a GET request in Jellycuts, retrieve a list of users in JSON format, and display their names.
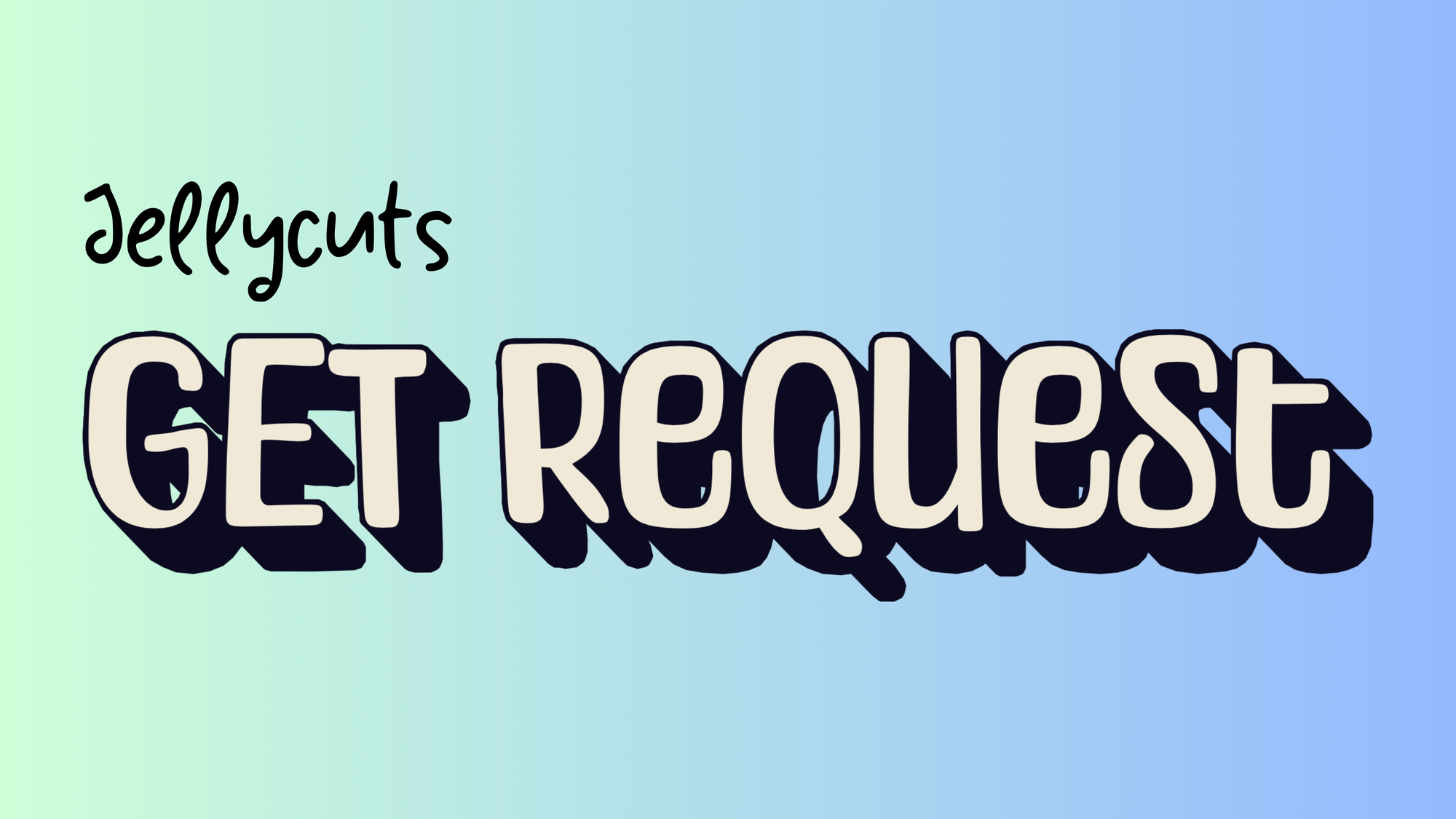
This article guides you through sending a GET request in Jellycuts to retrieve a list of users in JSON format and display their names.
I've organized the content into two sections for clarity:
- Complete Code with Explanation: This section presents the entire code and a brief explanation.
- Step-by-Step Instructions: This section breaks down the process into detailed steps.
Please leave your feedback in the comments below to let me know if you prefer having both sections or just one.
Full Example Code
Here's the complete Jellycuts script combining all the steps:
#Color: red
#Icon: shortcuts
import Shortcuts
// URL with 10 Users in JSON format
var cmsURL = "https://jsonplaceholder.typicode.com/users"
// GET Request
downloadURL(
url: cmsURL,
method: GET,
headers: {
"Content-Type": "application/json; charset=UTF-8",
"Accept": "application/json"
},
requestType: ,
requestJSON: {}
) >> response
// Loop
repeatEach(response) {
// Extract value from 'name' key
valueFor(key: "name", dictionary: RepeatItem) >> name
// Show each name
showResult(text: name)
}
// See Entire Array of Objects
quicklook(input: response)
Explanation
- URL and Headers: The URL points to a JSON resource. Headers specify the content type and desired response format.
- GET Request:
downloadURL
sends the GET request and stores the response in theresponse
variable. - Loop and Extract Data:
repeatEach
iterates through the response, extracting and displaying each user's name. - Display Response:
quicklook
shows the entire response for a complete overview.
Step-by-Step Guide
-
Set Up the URL and Import Shortcuts
Begin by defining the URL that points to the resource you want to fetch. For this example, we use a URL that returns a list of 10 users in JSON format. Import the Shortcuts library to access necessary functions.
#Color: red #Icon: shortcuts import Shortcuts
-
Define the URL
Define a variable for the URL that you will send the GET request to.
// URL with 10 Users in JSON format var cmsURL = "https://jsonplaceholder.typicode.com/users"
-
Send the GET Request
Use the
downloadURL
function to send a GET request. Specify the URL, method, headers, and request type. Since this is a GET request, there's no need to send a request body, sorequestJSON
is an empty dictionary.// GET Request downloadURL( url: cmsURL, method: GET, headers: { "Content-Type": "application/json; charset=UTF-8", "Accept": "application/json" }, requestType: , requestJSON: {} ) >> response
-
Process the Response
Loop through the response to extract and display specific data. In this example, we extract the value associated with the
name
key from each user object in the response.// Loop repeatEach(response) { // Extract value from 'name' key valueFor(key: "name", dictionary: RepeatItem) >> name // Show each name showResult(text: name) }
-
Display the Entire Response
Optionally, use the
quicklook
function to display the entire response for a comprehensive view of the data retrieved.// See Entire Array of Objects quicklook(input: response)