How to SEND a POST Request in Jellycuts
Creating POST requests in Jellycuts is a breeze, thanks to its intuitive execution sequence and powerful "downloadURL" method.
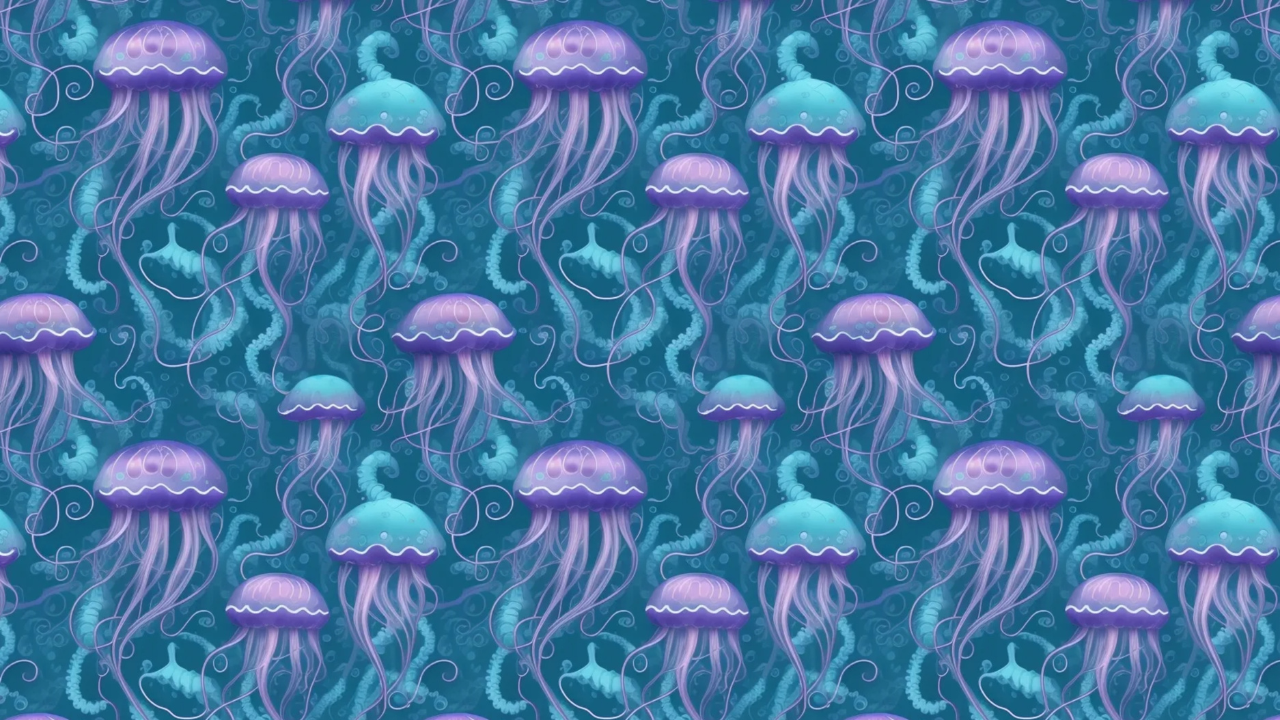
Creating POST requests can sometimes feel like a chore, but with Jellycuts, it’s a breeze! If you've been navigating the waters of Apple Shortcuts' "Get contents of" function, you'll find Jellycuts' "downloadURL" method refreshingly straightforward. Especially if you're already comfortable with JavaScript's Ajax, Swift's Alamofire, or JavaScript's Axios.
One of the standout features of Jellycuts is its intuitive execution sequence. Forget the tangled web of callbacks and async/await struggles—Jellycuts executes your code just as you write it, in a smooth, linear flow.
Let’s dive into an example to show just how simple it is to create a POST request in Jellycuts.
Here's a basic script to get you started:
import Shortcuts
#Color: red, #Icon: shortcuts
// This is a test API endpoint provided by JSONPlaceholder for creating and retrieving dummy post data, commonly used for prototyping and testing HTTP requests.
var cmsURL = "https://jsonplaceholder.typicode.com/posts"
// Arbitrary Variable
var num = 0.9
var json = { "title": "My First Post", "body": "${num}", "userId": 1 }
//
downloadURL(url: cmsURL, method: POST, headers: {"Content-Type": "application/json; charset=UTF-8"}, requestType: Json, requestJSON: json, requestVar: response) >> response
quicklook(input: response)
Let’s break it down:
import Shortcuts
: This imports the necessary Shortcuts framework.
#Color and #Icon
: These lines set the color and icon for your Jellycuts script—because why not make it look good too?
cmsURL
: The URL to which we're sending our POST request.
num
: An arbitrary variable, just to show how you can dynamically insert values.
json
: This is our JSON payload, formatted with the variable num.
downloadURL
: The magic method that handles the POST request. We specify the URL, method, headers, request type, and JSON payload here.
response
: This might stand out if you're coming from other programming languages. When you use >> in Jellycuts, you're creating a Magic Variable. A Magic Variable is immutable, meaning once it's set, it can't be changed. In this case, it elegantly handles the then/catch/error methods you'd typically see in other languages. So, instead of wrangling with those methods, you get a streamlined and efficient way to manage your responses.
quicklook
: Finally, we use this to display the response for a quick view.
See how clean and simple that is? No callback hell, no async headaches—just a straightforward POST request. Jellycuts makes it so easy, it almost feels like magic.